In the world of software development, efficiency and innovation reign supreme. Imagine a scenario where your team not only meets these demands but surpasses them, wielding AI as their secret weapon. This guide explores how integrating AI into the coding process is akin to having a sous-chef in the kitchen—a partnership that elevates the craft to gourmet levels.
Introduction: Why AI in Coding?
Imagine if your development team could transform into superheroes, with AI serving as their trusty sidekick. Just as a chef relies on a sous-chef to prep ingredients, allowing the main culinary artist to focus on creating that perfect dish, AI assists coders by setting up the coding elements. This enables the main ‘chef’—your developers—to concentrate on crafting gourmet code. For large organizations, staying ahead in the tech gastronomy means embracing AI coding principles, their secret spice rack, to streamline processes and innovate at scale.
The Essence of AI principles in Development
Your team is capable of doing great by applying these 7 principles in AI coding and starting from zero to master by understanding the principles. These will help leverage the existing knowledge and supercharge without having to go through hours of tedious sessions. These are the points I have formulated by personal experience and trial and errors for several months and perfecting the concepts in simplest form also known as AAA Principles for AI Coding.
Summary of the principles is published many months ago on GitHub( AI-Assisted-Programming-Principles ) and these are the practical and real world expanded explanation of the principles.
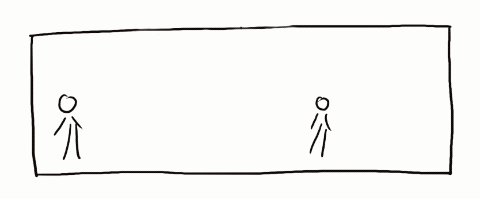
Principle 1: Intent of the Programmer
Like a GPS for a road trip, the intent guides you to your destination. It’s not about the specific turns you take but where you’re aiming to go. In large firms, a clear intent aligns diverse teams towards a common goal, focusing efforts on achieving specific outcomes and ensuring that every line of code contributes to the overarching vision.
(Code Examples added below for each principles. You can focus on AAA Core Concepts prior to code.)
Principle 2: Expression of Intent
Consider a dancer signaling their partner for the next move. In big companies, clarity in expressing intent helps AI follow the lead without missteps, ensuring that the development process is seamless and that AI tools are utilized to their fullest potential, enhancing efficiency and reducing errors.
Principle 3: Verbs and Nouns
Much like a well-organized tool shed, where hammers and saws (nouns) have clear purposes, and the actions we perform with them (verbs) are distinct, accurately labeling functions and variables helps AI understand the job at hand. This clarity in communication between human developers and AI tools is crucial for effective collaboration and maximizing the potential of AI in coding practices.
Principle 4: Multi-line Intents
Imagine a storyboard for a film. It outlines the scenes so the director knows what comes next. Similarly, multi-line intents give AI a clear narrative to follow, which is especially crucial when working on large-scale projects. This principle ensures that AI can accurately interpret and assist with complex coding tasks, facilitating a smoother development process.
Principle 5: Progression
Like a relay race where the baton is passed smoothly from one runner to the next, the code must progress from one stage to the next, a process that’s essential in complex organizational structures. This smooth progression allows for efficient coding practices, minimizing disruptions and ensuring that each component integrates seamlessly into the larger project.
Principle 6: Inputs and Expectations
Imagine a vending machine; you select a snack (input) and expect your choice to drop (output). Defining what you put into your code and what you expect out of it is crucial for AI to deliver the right ‘snack’. This principle emphasizes the importance of clear input and expected output definitions, enabling AI to more accurately assist in the coding process.
Principle 7: Validation
After a magician’s trick, the audience needs to see that the outcome is real, not an illusion. In the corporate world, validating AI’s suggestions ensures the magic is solid, executable code. This final principle highlights the necessity of rigorously testing and validating AI-generated code, ensuring its reliability and functionality within the project.
Practical Code Level Examples implementing the AAA Principles.
Before you begin to implement the code make sure you understand the easy 7 Core AAA principles above. Only examples are provided here. Full explanation provided above for each principle. It will be helpful for quick referencing and implementation.
Principle 1: Intent of the Programmer
Example: Function names that reflect their purpose.
// Incorrect: Unclear function purpose
function process(data) {
// Logic here
}
// Correct: Function name clearly states its intent
// Filters out non-numeric values from an array
function removeNonNumericValuesFromArray(values) {
return values.filter(value => !isNaN(value));
}
Principle 2: Expression of Intent
Example: Using variables that clearly state what they store.
// Incorrect: Vague variable name
let d = new Date().toISOString();
// Correct: Variable name explicitly expresses its content
// Stores the current date in ISO format
let currentDateInISO = new Date().toISOString();
Principle 3: Verbs and Nouns
Example: Clear naming for objects and their actions.
// Incorrect: Ambiguous class and method names
class DataHandler {
doWork(input) {}
}
// Correct: Class and method names are specific
// Class for validating user inputs
class UserInputValidator {
// Checks if the email address is valid
validateEmailAddress(email) {}
}
Principle 4: Multi-line Intents
Example: Structuring logic for readability and intent.
// Incorrect: All conditions cramped together
if (user.isActive && user.hasPermission("edit")) { editPost(); }
// Correct: Separating conditions for clarity
// Check if user is active
if (user.isActive) {
// Further check if user has edit permissions
if (user.hasPermission("edit")) {
editPost(); // User edits post
}
}
Principle 5: Progression
Example: Sequentially organizing code for logical flow.
// Incorrect: Disorganized execution order
logActivity("email sent", user); sendEmail(user); updateUserEmailCount(user);
// Correct: Order of operations reflects logical sequence
// Increment user's email count first
updateUserEmailCount(user);
// Log the email activity
logActivity("email sent", user);
// Finally, send the email
sendEmail(user);
Principle 6: Inputs and Expectations
Example: Documenting expected parameters and return values.
// Incorrect: No explanation of parameters or return value
function calculateDiscount(price) {}
// Correct: Document expected input and output
// Calculates discount based on provided price
// @param {Number} price - The original price of the item
// @return {Number} - The price after applying the discount
function calculateDiscount(price) {
// Discount logic here
return price - (price * 0.1); // Example: 10% discount
}
Principle 7: Validation
Example: Ensuring inputs meet expected criteria.
// Incorrect: No validation of inputs
function addUser(userData) {
database.save(userData);
}
// Correct: Validate userData before proceeding
// Validates user data before adding to database
function addUser(userData) {
if (userData && userData.name && userData.email) {
database.save(userData);
} else {
console.error("Invalid user data");
}
}
How to use this next?
For quick reference you can save all the information links above in your email and also forward to your team. Use the form below and signup to receive the links.
Once you signup, you’ll get –
1. Welcome email.
2. All the links and information of the AAA principles of AI coding in your email.
You can freely copy and distribute this to your team for referencing the principles of AI Coding.
By integrating these AAA AI coding principles, your large organization can streamline coding processes, ensuring that the implementation of AI tools and principles is as smooth as the gliding of a professional ice-skater across the rink. Embrace these principles, and transform your development team into the culinary masters of the tech world, where every line of code is a delicacy crafted to perfection.
There are also many companies who have studied their implementation and published white paper as well. You can read about measuring impacts and the and the white paper they published and its summary here – GenAI Tools in Large Organizations: Measuring Implementation Impact
Make sure to get the links on the email and bookmark for the team.
Comments
5 responses to “AI Coding vs AI Principles. Right strategy for Large Organizations to implement GenAI.”
After reading about AAA Principles, I’m convinced my coffee maker could code better than me with AI. 😂
Until AI can debug itself, I’ll keep my manual coding skills sharp. Just in case…
Curious how this meshes with legacy systems. Anyone tried integrating AI principles with older codebases?
David, Yes. I have tried them and referencing to the functions in a conversational comment does help grab the input args required and does a pretty good job.
[…] can also read about the ‘AAA Principles’ for AI coding which gives you a better understanding of how interaction with GenAI can be implemented in just 7 […]